Controls
Checkbox & Toggle
Checkbox and toggle controls let you manage boolean (true/false) values or multiple choices in your blocks. Both controls provide similar functionality with different visual appearance.
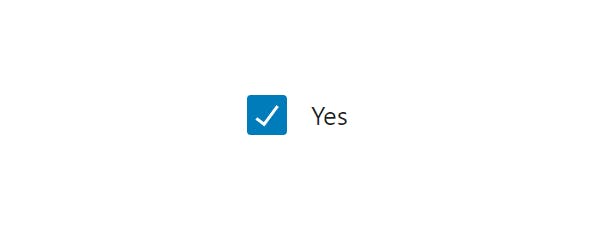
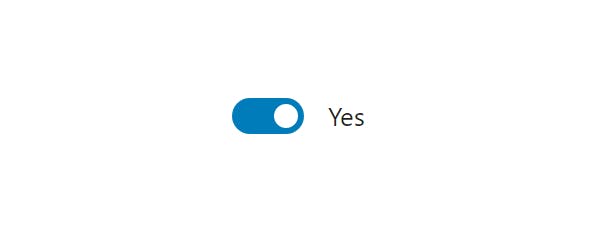
Control Settings
Single Checkbox/Toggle
- Alongside Text - Text displayed next to the control
- Checked - Set the default checked state
Multiple Checkboxes
When Multiple option is enabled, the following settings are available:
- Alongside Text - Text displayed next to the control
- Multiple - Enable multiple checkboxes
- Choices - Add options for multiple checkboxes
- Output Format - Specifies the returned value format. Choose from
Value
,Label
orBoth (Array)
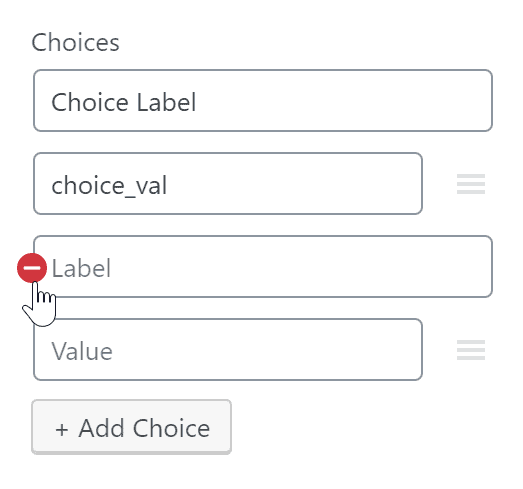
Usage
Single Checkbox/Toggle
<?php if ( $attributes['control_name'] ) : ?>
<p>The value is True</p>
<?php else: ?>
<p>The value is False</p>
<?php endif; ?>
{{#if control_name}}
<p>The value is True</p>
{{else}}
<p>The value is False</p>
{{/if}}
Multiple Checkboxes
<?php
// Returns array of selected values
foreach ( $attributes['control_name'] as $value ) {
echo '<p>' . esc_html( $value ) . '</p>';
}
?>
<?php
// Returns array of selected labels
foreach ( $attributes['control_name'] as $label ) {
echo '<p>' . esc_html( $label ) . '</p>';
}
?>
<?php
// Returns array of arrays with value and label
foreach ( $attributes['control_name'] as $choice ) {
echo '<p>Value: ' . esc_html( $choice['value'] ) . '</p>';
echo '<p>Label: ' . esc_html( $choice['label'] ) . '</p>';
}
?>
{{#each control_name}}
<p>{{this}}</p>
{{/each}}
{{#each control_name}}
<p>{{this}}</p>
{{/each}}
{{#each control_name}}
<p>Value: {{this.value}}</p>
<p>Label: {{this.label}}</p>
{{/each}}
Post Meta
<?php if ( get_lzb_meta( 'control_meta_name' ) ) : ?>
<p>The value is True</p>
<?php else: ?>
<p>The value is False</p>
<?php endif; ?>
<?php
$selected_choices = get_lzb_meta( 'control_meta_name' );
// Output depends on selected Output Format
foreach ( $selected_choices as $choice ) {
echo '<p>' . esc_html( $choice ) . '</p>';
}
?>
When Multiple option is enabled, the control returns an array of selected choices. The format of the array items depends on the Output Format setting.