Controls
Taxonomy
Taxonomy control lets you search and select terms from any WordPress taxonomy (categories, tags, or custom taxonomies).
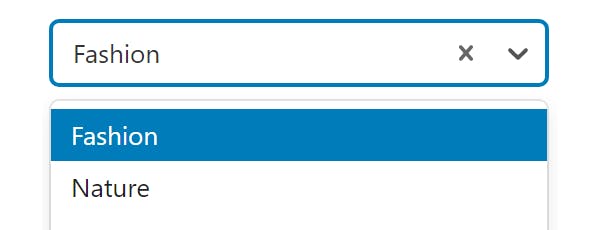
Only taxonomies registered with show_in_rest => true
will appear in the control. If your custom taxonomy is not showing up, add this parameter:
register_taxonomy('your_taxonomy', 'post', [
// ... other parameters
'show_in_rest' => true,
]);
Control Settings
- Taxonomy - Choose taxonomy type (Categories, Tags, etc.)
- Appearance - Display as
Select
,Checkbox
, orRadio
- Output Format - Choose data format:
Term Slug
- Returns term slugTerm ID
- Returns term IDTerm Object
- Returns full term object
- Multiple - Allow selecting multiple terms
Usage Examples
Basic Usage (Term Slug)
<?php
$term = get_term_by('slug', $attributes['control_name'], 'category');
if ($term && !is_wp_error($term)) {
echo '<span class="term">' . esc_html($term->name) . '</span>';
}
?>
Multiple Terms
<?php if ($attributes['control_name']) : ?>
<ul class="terms-list">
<?php foreach($attributes['control_name'] as $term_slug) :
$term = get_term_by('slug', $term_slug, 'category');
if ($term && !is_wp_error($term)) : ?>
<li class="term-<?php echo esc_attr($term->slug); ?>">
<?php echo esc_html($term->name); ?>
</li>
<?php endif;
endforeach; ?>
</ul>
<?php endif; ?>
Using Term Object
<?php if ($attributes['control_name']) : ?>
<div class="term-<?php echo esc_attr($attributes['control_name']->slug); ?>">
<h4><?php echo esc_html($attributes['control_name']->name); ?></h4>
<p><?php echo esc_html($attributes['control_name']->description); ?></p>
</div>
<?php endif; ?>
Handlebars Usage
Set Output Format to Term Object
when using Handlebars templates.
<div class="term-{{control_name.slug}}">
{{control_name.name}}
</div>
<ul class="terms-list">
{{#each control_name}}
<li class="term-{{this.slug}}">
{{this.name}}
</li>
{{/each}}
</ul>
Post Meta
<?php
$term_slug = get_lzb_meta('control_meta_name');
if ($term_slug) {
$term = get_term_by('slug', $term_slug, 'category');
if ($term && !is_wp_error($term)) {
echo '<span class="term">' . esc_html($term->name) . '</span>';
}
}
?>
Use Term Object format to access all term data without additional database queries.